Aggregation - Part 14
- JP
- May 11, 2015
- 2 min read
Aggregations are special types of operations that essentially process several data records and return only the computed results. Now essentially, they’re a method to give you just the results from the operation. That can be contrasted with the find or read operations we looked at in previous videos, which bring back a whole set of records, aggregations only bring you back the aggregate results. Now this has a lot of advantages. It can certainly simplify the application code, and it can limit resource utilization. If I’m returning 1,000 records, well that obviously takes some level of resources, RAM, and processor, and that sort of thing, but if I’m simply returning, for example, the count of those records, well then that takes a lot less resources. There’s several ways to do an aggregation, there are basically three primary ways that get used. Aggregations are operations that process data records and return completed results. Aggregation operations are used to perform calculations on data sets. They can simplify application code and limit resource utilization. Aggregation modalities include using
Aggregation pipelines – documents enter a multi-stage pipeline and are transformed into an aggregated result.
Map-Reduce programming – Map-Reduce programming typically has two phases. The map stage processes each document and emits one or more objects. The reduce stage combines the output of the map operation. During an optional finalize stage, final modifications can be made to the result.
Single-purpose aggregation operations – provide simple access to common aggregation processes.
use aggregation pipeline operations to transform documents
Transforming documents
In MongoDB, the pipeline is a way to refer to the data that you’re producing for you to work with. Now, we might want to limit or to adjust or to sort, or to do all sorts of things with that pipeline. In the past, you have seen the limit() function in some previous videos. Let’s briefly review that, then show you some new functions. db.products.find(), that’s going to return all the records as you see there. Or I can choose db.products.find().limit(), and put in any number smaller than the total, let’s say 5. Now I only get the first five records, starting with the first one going to the fifth. Well we have another option we can do with that, we can skip something. db.products.find().limit(), let’s say 5 again, but skip the first one. You notice I still get five, but we skipped the first and started from second onward. That may be useful to you when you need to traverse various records.
db.products.find().limit(5)

db.products.find().limit(5).skip(1)

we can also perform sorting on any field
db.products.find().sort({brand:1})
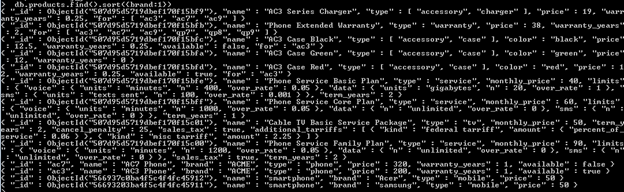
sorting on multiple fields
db.products.find().sort({brand:1, price:1})
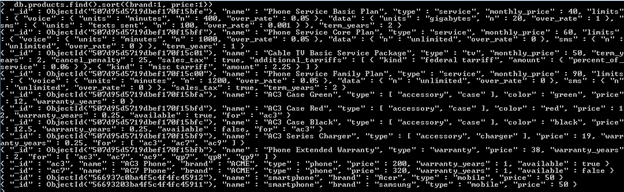
It’s also possible to use aggregate functions in order to look for a matching record. Let’s try this: db.products.aggregate(), and any time you use aggregate() you pass in an array, so we have square brackets, open my braces, because this is JSON format, and I use the word $match. I would like to get items that match a particular criteria. Open brackets, and let’s say, brand: “Acer”.
db.products.aggregate([{$match : { brand:”Acer”}}])
